Equal Beauty CodeChef SnackDown 2021 Round 1A Question The beauty of an (non-empty) array of integers is defined as the difference between its largest and smallest element. For example, the beauty of the array [2,3,4,4,6] is 6−2=4. An array A is said to be good if it is possible to partition the elements of A into two non-empty arrays B1 and B2 such that B1 and B2 have the same beauty. Each element of array A should be in exactly one array: either in B1 or in B2. For example, the array [6,2,4,4,4] is good because its elements can be partitioned into two arrays B1=[6,4,4] and B2=[2,4], where both B1 and B2 have the same beauty (6−4=4−2=2). You are given an array A of length N. In one move you can: Select an index i (1≤i≤N) and either increase Ai by 1 or decrease Ai by 1. Find the minimum number of moves required to make the array A good. Input Format The first line of input contains a single integer T, denoting the number of test cases. The description of T test cases follow. Each test
Split Linked List in Parts LeetCode Solution
Question
Given the head
of a singly linked list and an integer k
, split the linked list into k
consecutive linked list parts.
The length of each part should be as equal as possible: no two parts should have a size differing by more than one. This may lead to some parts being null.
The parts should be in the order of occurrence in the input list, and parts occurring earlier should always have a size greater than or equal to parts occurring later.
Return an array of the k
parts.
Example 1:
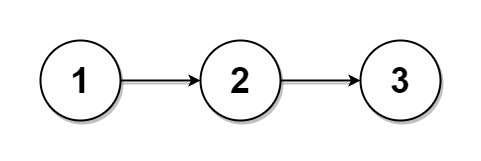
Input: head = [1,2,3], k = 5 Output: [[1],[2],[3],[],[]] Explanation: The first element output[0] has output[0].val = 1, output[0].next = null. The last element output[4] is null, but its string representation as a ListNode is [].
Example 2:

Input: head = [1,2,3,4,5,6,7,8,9,10], k = 3 Output: [[1,2,3,4],[5,6,7],[8,9,10]] Explanation: The input has been split into consecutive parts with size difference at most 1, and earlier parts are a larger size than the later parts.
Constraints:
- The number of nodes in the list is in the range
[0, 1000]
. 0 <= Node.val <= 1000
1 <= k <= 50
Explanation
The question is of medium category of LeetCode. To solve this problem you need a clear idea about linked list and some knowledge regarding splitting of linked list.Firstly, we need to get length of the linked list start with head node, let say listSize. We need to split into k parts, where the length of each part should be as equal as possible: Each part have the size, partSize = listSize / k. When dividing into k parts, there may have some remain elements, remainSize = listSize % k. We need to prioritize filling those remaining elements into earlier parts since the problem said: parts occurring earlier should always have a size greater than or equal to parts occurring later. The time complexity of this code is O(N) and the space complexity of this code is O(1). That's all now code it. Hope you understood the explanation. I recommend to try the problem by yourself first then you can have a look at the solution given below in Java, C++, Python.
More information about LeetCode Daily Challenge
More Information About LeetCode Daily Challenge
LeetCode is a great coding platform which provides daily problems to solve and maintain a streak of coding. This platform helps in improvement of coding skills and attempting the daily challenge makes our practice of coding regular. With its bunch of questions available it is one of the best coding platforms where you can have self improvement. LeetCode also holds weekly and biweekly contests which is a great place to implement you knowledge and coding skills which you learnt through out the week by performing the daily challenge. It also provides badges if a coder solves all the daily problems of a month. For some lucky winners T-shirts are also given and that too for free. If you get the pro version you can get more from LeetCode like questions that are asked in interviews, detailed explanation of each chapter and each concept and much more. LeetCode challenges participants with a problem from our carefully curated collection of interview problems every 24 hours.
All users with all levels of coding background are welcome to join!
@PREMIUM USERS will have an extra carefully curated premium challenge weekly and earn extra LeetCoin rewards. The premium challenge will be available together with the first October challenge of the week and closed together with the November challenge of the week.
Starting from 2021, users who completed all Daily LeetCoding Challenge non-premium problems for the month (with or without using Time Travel Tickets) will win a badge to recognize their consistency! Your badges will be displayed on your profile page. Completing each non-premium daily challenge. (+10 LeetCoins)
Completing 25 to 30 non-premium daily challenges without using Time Travel Ticket will be eligible for an additional 25 LeetCoins. (Total = 275 to 325 LeetCoins)
Completing all 31 non-premium daily challenges without using Time Travel Ticket will be eligible for an additional 50 LeetCoins, plus a chance to win a secret prize ( Total = 385 LeetCoins + *Lucky Draw)!
Lucky Draw: Those who complete all 31 non-premium daily challenges will be automatically entered into a Lucky Draw, where LeetCode staff will randomly select 3 lucky participants to each receive one LeetCode Polo Shirt on top of their rewards! Do you have experience trying to keep a streak but failed because you missed one of the challenges?
Hoping you can travel back to the missed deadline to complete the challenge but that's not possible......or is it?
With the new Time Travel Ticket , it's possible! In order to time travel, you will need to redeem a Time Travel Ticket with your LeetCoins. You can redeem up to 3 tickets per month and the tickets are only usable through the end of the month in which they are redeemed but you can use the tickets to make up any invalid or late submission of the current year. To join, just start solving the daily problem on the calendar of the problem page. You can also find the daily problem listed on the top of the problem page. No registration is required. The daily problem will be updated on every 12 a.m. Coordinated Universal Time (UTC) and you will have 24 hours to solve that challenge. Thar's all you needed to know about Daily LeetCode Challenge.
Program Code
Java
class Solution
{
public ListNode[] splitListToParts(ListNode head, int k)
{
ListNode temp = head;
int size = 0;
while(temp != null)
{
size++;
temp = temp.next;
}
ListNode ans[] = new ListNode[k];
int s[] = new int[k];
int c = 0;
while(size-- > 0)
{
s[c % k]++;
c++;
}
temp = head;
int idx = 0;
for(int num : s)
{
ListNode hh = null;
ListNode tt = null;
while(num-- > 0)
{
if(tt == null)
{
hh = new ListNode(temp.val);
tt = hh;
}
else
{
tt.next = new ListNode(temp.val);
tt = tt.next;
}
temp = temp.next;
}
ans[idx++] = hh;
}
return ans;
}
}
C++
class Solution {
public:
vector<ListNode*> splitListToParts(ListNode* head, int k) {
int ListLen = 0, eachLen = 0, remain = 0, size = 0, len = 0;
ListNode *cur = NULL, *prev = NULL, *newHead = NULL;
/* Init answer vector<ListNode*> to k NULL lists. */
vector<ListNode*> ans = vector<ListNode*>(k, NULL);
/* Get input list length. */
cur = head;
while(cur != NULL) {
ListLen++;
cur = cur->next;
}
eachLen = ListLen / k;
remain = (ListLen % k);
cur = head;
newHead = head;
/* Assign the remain to the first few lists */
len = eachLen + (remain > 0 ? 1 : 0);
remain--;
while(cur != NULL) {
if (--len == 0) {
prev = cur;
cur = cur->next;
prev->next = NULL;
ans[size++] = newHead;
newHead = cur;
len = eachLen + (remain > 0 ? 1 : 0);
remain--;
} else {
cur = cur->next;
}
}
return ans;
}
};
Python
class Solution:
def splitListToParts(self, root, k):
cur = root
N = 0
while cur:
cur = cur.next
N += 1
d, r = divmod(N, k)
ans = []
cur = root
for i in range(k):
head = cur
for j in range(d + (i < r) - 1):
if cur: cur = cur.next
if cur:
cur.next, cur = None, cur.next
ans.append(head)
return ans
Comments
Post a Comment