Equal Beauty CodeChef SnackDown 2021 Round 1A Question The beauty of an (non-empty) array of integers is defined as the difference between its largest and smallest element. For example, the beauty of the array [2,3,4,4,6] is 6−2=4. An array A is said to be good if it is possible to partition the elements of A into two non-empty arrays B1 and B2 such that B1 and B2 have the same beauty. Each element of array A should be in exactly one array: either in B1 or in B2. For example, the array [6,2,4,4,4] is good because its elements can be partitioned into two arrays B1=[6,4,4] and B2=[2,4], where both B1 and B2 have the same beauty (6−4=4−2=2). You are given an array A of length N. In one move you can: Select an index i (1≤i≤N) and either increase Ai by 1 or decrease Ai by 1. Find the minimum number of moves required to make the array A good. Input Format The first line of input contains a single integer T, denoting the number of test cases. The description of T test cases follow. Each ...
Path Sum III LeetCode Solution
Question
Given the root
of a binary tree and an integer targetSum
, return the number of paths where the sum of the values along the path equals targetSum
.
The path does not need to start or end at the root or a leaf, but it must go downwards (i.e., traveling only from parent nodes to child nodes).
Example 1:
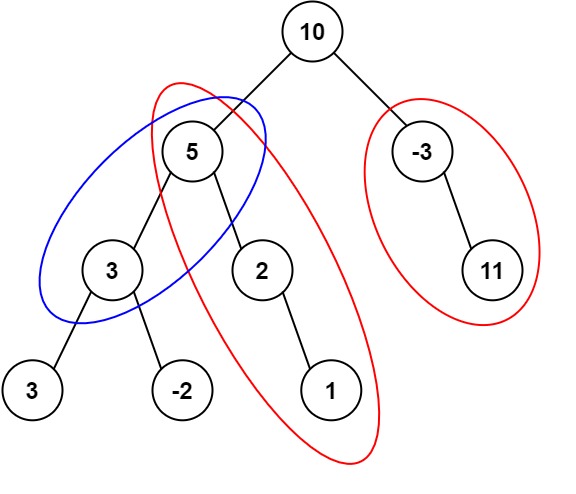
Input: root = [10,5,-3,3,2,null,11,3,-2,null,1], targetSum = 8 Output: 3 Explanation: The paths that sum to 8 are shown.
Example 2:
Input: root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22 Output: 3
Constraints:
- The number of nodes in the tree is in the range
[0, 1000]
. -109 <= Node.val <= 109
-1000 <= targetSum <= 1000
Explanation
This is a good question to test your logical skills. This question is categorized as medium on LeetCode. Lets know how to solve it by the detailed explanation given here. We can do it by recursion only but it is to much time consuming so to reduce the time complexity we are storing a precomputed sum and their corresponding frequencies in a Hash map. The concept which we are using here is Depth First Search (DFS) by preorder traversal. This question is similar to subarray sum equals target k. The idea is that at each node update a hash of previous prefixes and a prefix sum; and look at the previous prefixes that differ to prefix sum by k. One small addition to this is when a node and its children are fully visited we need to undo the changes in hash and prefix sums before retreating to the parent in a previous level of recursion. The time complexity and space complexity both will be O(N) where N is the total number of nodes in the tree. That's it now code it , hope you understood the explanation well. I recommend to try the problem by yourself first by following the above explanation but if you are stuck then you can visit the solution given below in Java, C++, Python3.
Program Code
Java
class Solution {
int pathCnt = 0;
Map<Integer, Integer> preMap = new HashMap<>();
public int pathSum(TreeNode root, int targetSum) {
int preSum = 0;
preMap.put(0, 1);
dfs(root, targetSum, preSum);
return pathCnt;
}
private void dfs(TreeNode root, int targetSum, int preSum){
if(root == null){
return;
}
preSum += root.val;
if(preMap.containsKey(preSum - targetSum)){
pathCnt += preMap.get(preSum - targetSum);
}
// increament the currentPreSum freq in map
preMap.put(preSum, preMap.getOrDefault(preSum, 0)+1);
// recurse for the left and right subtree
dfs(root.left, targetSum, preSum);
dfs(root.right, targetSum, preSum);
// here is a litte detail to notice:::: at the end the preSent value of preSum would be
//
moving out of the recursion stack thus we are decreamenting it's freq in the hashmap
if(preMap.get(preSum) - 1 > 0)
preMap.put(preSum, preMap.getOrDefault(preSum, 0)-1);
else
preMap.remove(preSum);
}
}
C++
class Solution {
public:
int dfs( TreeNode* root , unordered_map<int,int>& umap , int sum , int target){
int count = 0;
sum += root->val;
int extra = sum - target ;
if( umap.find(extra) != umap.end() ){
count += umap[extra];
}
int a = 0 , b = 0 ;
umap[sum]++;
if( root->left){
a = dfs(root->left,umap,sum,target);
}
if( root->right){
b = dfs( root->right,umap,sum, target );
}
umap[sum]--;
return a+b+count;
}
int pathSum(TreeNode* root, int targetSum) {
if( root==NULL ){
return 0;
}
unordered_map<int,int> umap;
umap[0] = 1 ;
return dfs(root,umap,0,targetSum);
}
};
Python3
class Solution:
def pathSum(self, root: Optional[TreeNode], targetSum: int) -> int:
count=0
prefixsums=collections.defaultdict(int)
prefixsums[0]=1 #initialize dictionary at empty prefix
prefixsum=0
def dfs(node):
if not node:
return
nonlocal count
nonlocal prefixsum
prefixsum+=node.val
count+=prefixsums[prefixsum-targetSum]
prefixsums[prefixsum]+=1
dfs(node.left)
dfs(node.right)
prefixsums[prefixsum]-=1
prefixsum-=node.val
dfs(root)
return count
More Information About LeetCode Daily Challenge
LeetCode is a great coding platform which provides daily problems to solve and maintain a streak of coding. This platform helps in improvement of coding skills and attempting the daily challenge makes our practice of coding regular. With its bunch of questions available it is one of the best coding platforms where you can have self improvement. LeetCode also holds weekly and biweekly contests which is a great place to implement you knowledge and coding skills which you learnt through out the week by performing the daily challenge. It also provides badges if a coder solves all the daily problems of a month. For some lucky winners T-shirts are also given and that too for free. If you get the pro version you can get more from LeetCode like questions that are asked in interviews, detailed explanation of each chapter and each concept and much more. LeetCode challenges participants with a problem from our carefully curated collection of interview problems every 24 hours.
All users with all levels of coding background are welcome to join!
@PREMIUM USERS will have an extra carefully curated premium challenge weekly and earn extra LeetCoin rewards. The premium challenge will be available together with the first October challenge of the week and closed together with the November challenge of the week.
Starting from 2021, users who completed all Daily LeetCoding Challenge non-premium problems for the month (with or without using Time Travel Tickets) will win a badge to recognize their consistency! Your badges will be displayed on your profile page. Completing each non-premium daily challenge. (+10 LeetCoins)
Completing 25 to 30 non-premium daily challenges without using Time Travel Ticket will be eligible for an additional 25 LeetCoins. (Total = 275 to 325 LeetCoins)
Completing all 31 non-premium daily challenges without using Time Travel Ticket will be eligible for an additional 50 LeetCoins, plus a chance to win a secret prize ( Total = 385 LeetCoins + *Lucky Draw)!
Lucky Draw: Those who complete all 31 non-premium daily challenges will be automatically entered into a Lucky Draw, where LeetCode staff will randomly select 3 lucky participants to each receive one LeetCode Polo Shirt on top of their rewards! Do you have experience trying to keep a streak but failed because you missed one of the challenges?
Hoping you can travel back to the missed deadline to complete the challenge but that's not possible......or is it?
With the new Time Travel Ticket , it's possible! In order to time travel, you will need to redeem a Time Travel Ticket with your LeetCoins. You can redeem up to 3 tickets per month and the tickets are only usable through the end of the month in which they are redeemed but you can use the tickets to make up any invalid or late submission of the current year. To join, just start solving the daily problem on the calendar of the problem page. You can also find the daily problem listed on the top of the problem page. No registration is required. The daily problem will be updated on every 12 a.m. Coordinated Universal Time (UTC) and you will have 24 hours to solve that challenge. Thar's all you needed to know about Daily LeetCode Challenge.
Comments
Post a Comment